Displaying reports on a form might be a good thing on its own, but do a modicum of research and you will see that in real world reports are more likely to be standalone files – .pdf, .xls, or other.
Because, as some say, sharing is sexy

In this post, I’ll quickly show how you can export a SharpShooter report into a handful of different formats:
- Excel
- CSV
- HTML
- RTF
- XPS
Step 1 of 2. Prepare.
Let’s start from the beginning! We open up Visual Studio, create a new WinForms application, drag-n-drop a ReportManager on the main form, and then create an InlineReportSlot for our report manager (don’t need anything fancy in this example, so storing report layout details within Form1.resx is just fine).
Then we doubleclick on the report slot to open up Report Designer, and concoct the following masterpiece:
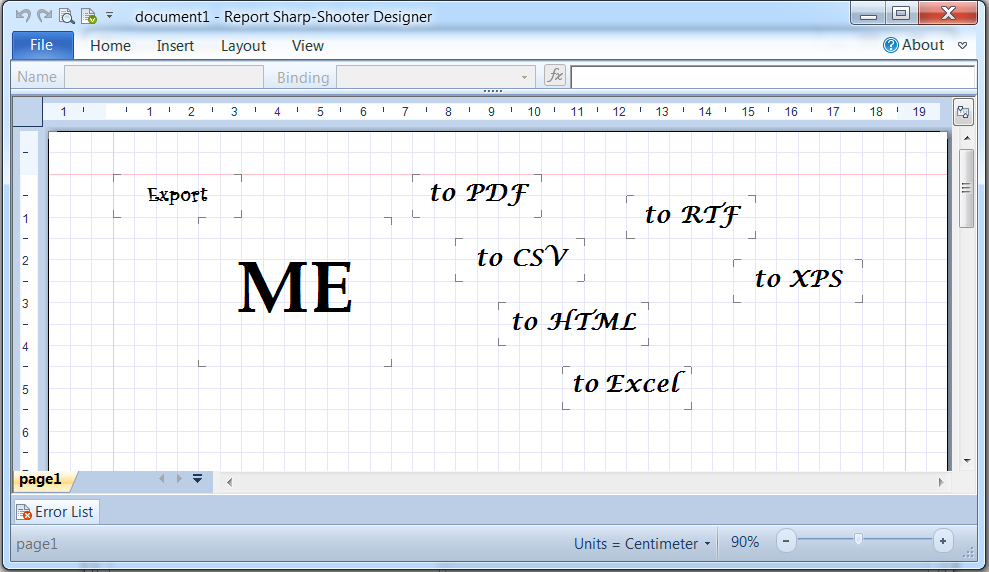
That is it – we don’t use any “real data” and will just export the report, to keep the example simple.
Step 2 of 2. Implement report export.
This part is even easier, since diligent SharpShooter developers already wrote (almost) all the code. Here’s our main form and button handlers:
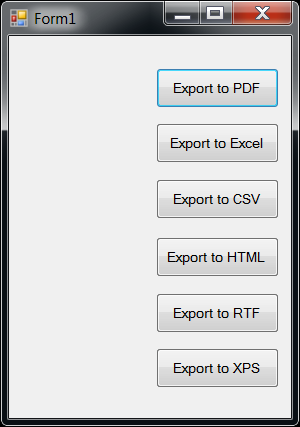
private void btnExportPdf_Click(object sender, EventArgs e) { reportSlot.ExportTo(@"C:\Temp\doc1.pdf"); } private void btnExportExcel_Click(object sender, EventArgs e) { reportSlot.ExportTo(@"C:\Temp\doc1.xls"); } ... private void btnExportXps_Click(object sender, EventArgs e) { reportSlot.ExportTo(@"C:\Temp\doc1.xps"); }
Can’t you believe it is that simple? Oh well, it’s not – there’s no ExportTo on ReportSlot class. I created it as an extension method, to keed the code clean. Below is the implementation:
internal static class ExportExtensions { internal static void ExportTo(this ReportSlot slot, string file) { ExportFilter filter; switch (Path.GetExtension(file)) { case ".pdf": filter = new PdfExportFilter(); break; case ".xls": filter = new ExcelExportFilter(); break; case ".csv": filter = new CsvExportFilter(); break; case ".html": filter = new HtmlExportFilter(); break; case ".rtf": filter = new RtfExportFilter(); break; case ".xps": filter = new XpsExportFilter(); break; default: throw new ArgumentOutOfRangeException( "file", "Cannot export to " + Path.GetExtension(file) ); } filter.Export(slot.RenderDocument(), file, false); } }
Now that’s truly it. One thing to note is the last boolean parameter in filter.Export – it describes whether we want an “Export Settings” dialog to be displayed. As I haven’t found a compelling reason to display the dialog, I decided to pass false and make all export operations silent.
Below you can see the HTML version (and others look great too!):
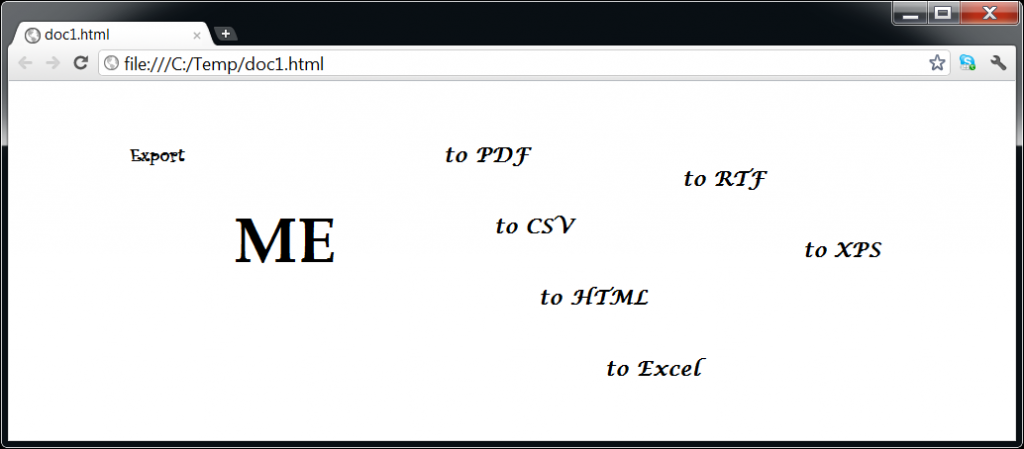
Summary.
Export SharpShooter reports to PDF, Excel, CSV, HTML, RTF, and XPS proved to be absurdly easy – you just need to use an appropriate ExportFilter, and SharpShooter will do the rest.
As usual, you can browse source code of this example or download it a a zip archive.
Happy reporting!