I have a confession to make: I don’t like using Visual Studio when I play with Report SharpShooter.
Because for my tasks it’s ridiculous. Create a WinForms app, drop ReportManager on it, add a report slot, setup “Preview” button, and so on, on and on – all this rigamarole of the same steps every time I need to preview a report. Or design a new one.
There must be a better way, I thought. A way that would allow to bypass Visual Studio altogether, and display a report (or bring up Report Designer) immediately when you need it. And in this very article, my dear readers, I’m proudly presenting to you this new fascinating way of working with Sharp-Shooter.
Powershell.
We will be using PowerShell, a command line shell from Microsoft. Without getting too deep, it’s a scripting language built on top of .NET framework, it is totally free and comes preinstalled on Windows7 and a few others, and if you don’t have it then here’s a download page.
Powershell allows us to do pretty much everything we can do with C#, although in a somewhat less convenient way (which is probably why there are no plans to rewrite SharpShooter in powershell). But it comes in handy for small scripts, it eliminates the “human factor” errors, it can be run from a command line, and it can be edited in Notepad. What else can one dare to dream of!
Working with reports from Powershell.
Powershell uses a verb-noun pair for the names of its commands, and we’d be following this guideline. I’ll share the full code in the next section, and now just want to show how you can display a report from powershell console:
Show-Report "C:\Reports\some.rst"
Nothing else. No Visual Studio and no code – just the above line and you’ll get a preview for your some.rst report. The same for designing a new report: New-Report command will bring up Report Designer.
To make it even more clear, below is a walkthrough of everything I’ve done to create a report and display it.
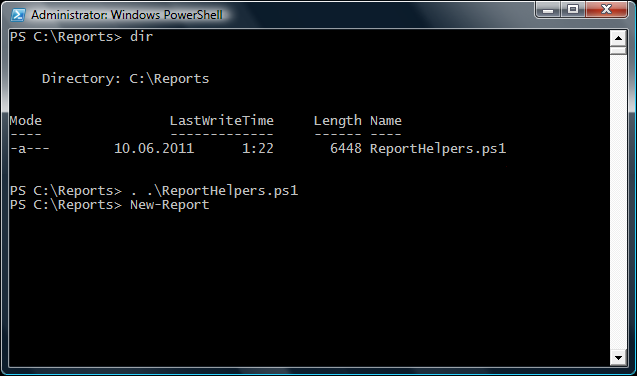
The first command dir displays the content of the current folder: there’s only one powershell file, which contains the functions we need.
The second command . .\ReportHelpers.ps1 (notice, it’s dot space dot backslash and file name) includes this powershell script into the current context, and from now on we can use the functions defined in that file.
The third command New-Report opens up Report Designer (takes about 3-5 seconds on my laptop). In Report Designer, I create a new blank report, drop an image on it and save the result:
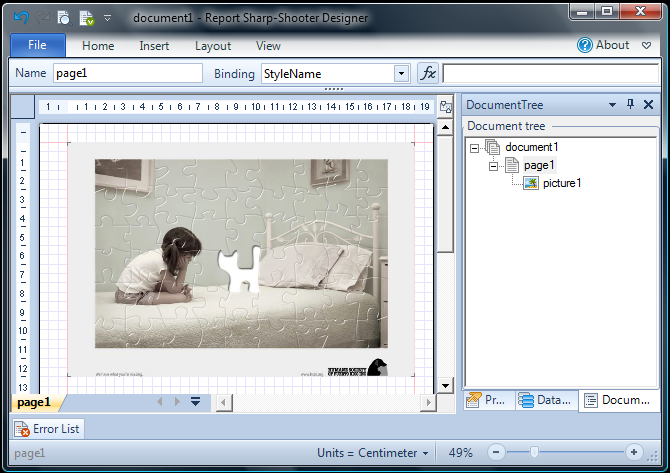
Then I go back to powershell console, hit dir again (just to show you the .rst file has appeared) and run the preview with Show-Report:
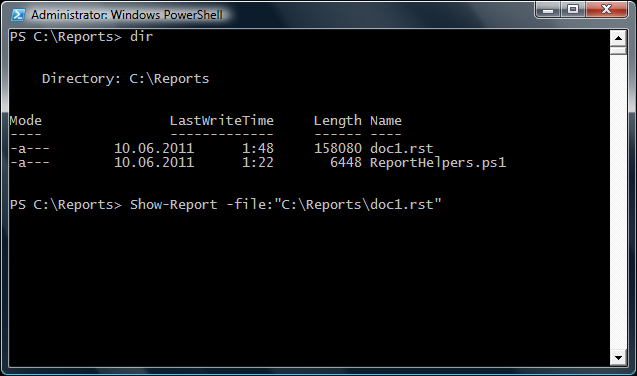
..and happily get the preview:
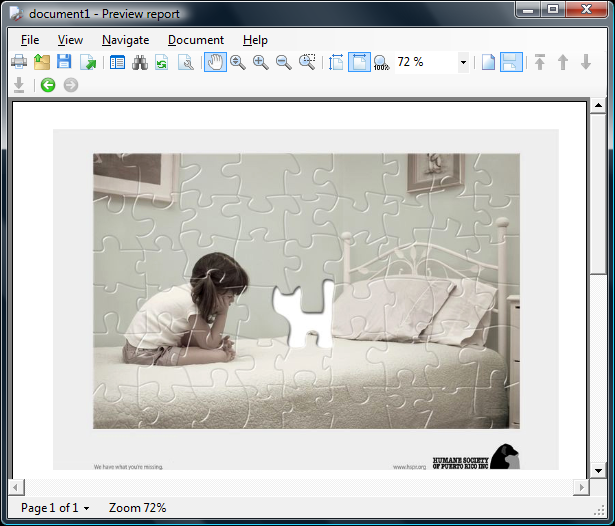
How this works.
First off, feel free to browse the script on BitBucket and learn how it works. Patches and suggestions are welcome
And below I’ll go through the most interesting parts.
- Report SharpShooter cannot work without a WinForm, so we need to create a hidden form in powershell
$hiddenForm = new-object System.Windows.Forms.Form $hiddenForm.ShowInTaskbar = $false $hiddenForm.Size = new-object System.Drawing.Size(1, 1)
- Creating a FileReportSlot in powershell seems easy, although syntax for adding event handlers is not straightforward:
$slot = new-object PerpetuumSoft.Reporting.Components.FileReportSlot $slot.FilePath = $file $slot.add_RenderCompleted({ _displayGeneratedReport })
- When our handler gets invoked, we need to close the hidden form, to prevent memory leaks:
function _displayGeneratedReport() { if ($hiddenForm -ne $null) { $hiddenForm.Close() } ... }
- SharpShooter needs to run in “Single Threaded Apartment” mode (this is a topic in itself, but without getting into a huge discussion about threading, it needs STA because WinForms are not thread-safe). So we have to wrap the invocation into an STA call, because Powershell runs in MTA by default:
function New-Report() { powershell -noprofile -sta -command { ... $slot = new-object PerpetuumSoft.Reporting.Components.FileReportSlot $slot.FilePath = "Temp.rst" $slot.DesignTemplate() } }
Summary.
As we have just seen, PowerShell is a decent alternative to Visual Studio when it comes to working with SharpShooter reports. Editing powershell scripts can be, at times, faster that kicking off Visual Studio and coding there – and surely a powershell script is a lot more transparent that a compiled .NET application.
The commands we tried in this article are generic enough to be reused without modification – and I bet there’s a lot more we can do here. Stay tuned!